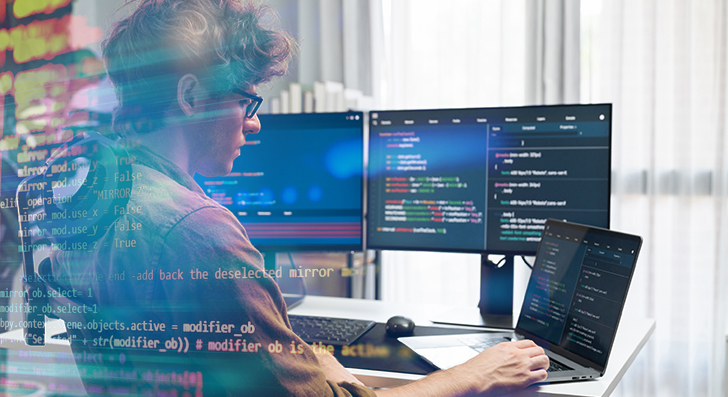
Scalability suggests your application can manage development—more people, far more data, and much more traffic—without the need of breaking. Being a developer, creating with scalability in your mind saves time and stress afterwards. Here’s a transparent and sensible guideline to assist you to commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability is not a little something you bolt on afterwards—it should be section of the plan from the beginning. Many apps are unsuccessful whenever they expand fast due to the fact the original layout can’t handle the extra load. To be a developer, you should Imagine early about how your system will behave under pressure.
Get started by developing your architecture to generally be flexible. Keep away from monolithic codebases where every little thing is tightly related. Alternatively, use modular design or microservices. These patterns split your application into smaller, independent areas. Each individual module or support can scale By itself without having influencing The complete method.
Also, think of your databases from working day 1. Will it want to manage one million consumers or merely 100? Pick the correct sort—relational or NoSQL—determined by how your details will grow. Strategy for sharding, indexing, and backups early, even if you don’t have to have them yet.
An additional crucial position is to stop hardcoding assumptions. Don’t generate code that only works under present situations. Give thought to what would materialize if your user foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use layout designs that assist scaling, like information queues or celebration-pushed programs. These support your app take care of far more requests with no receiving overloaded.
If you Establish with scalability in your mind, you're not just getting ready for success—you're lessening upcoming problems. A perfectly-prepared technique is less complicated to keep up, adapt, and increase. It’s far better to prepare early than to rebuild afterwards.
Use the ideal Databases
Selecting the right databases is a vital Section of creating scalable applications. Not all databases are built the exact same, and using the Incorrect you can sluggish you down or even trigger failures as your application grows.
Start off by knowing your data. Could it be extremely structured, like rows inside of a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient in shape. They're strong with associations, transactions, and consistency. Additionally they assistance scaling approaches like go through replicas, indexing, and partitioning to take care of a lot more traffic and data.
If the info is a lot more flexible—like consumer activity logs, merchandise catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at handling massive volumes of unstructured or semi-structured data and may scale horizontally extra very easily.
Also, take into consideration your go through and produce patterns. Have you been accomplishing a great deal of reads with much less writes? Use caching and read replicas. Do you think you're managing a hefty publish load? Take a look at databases that may take care of superior write throughput, or maybe event-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also wise to Assume in advance. You might not need Sophisticated scaling capabilities now, but deciding on a databases that supports them means you won’t require to switch later.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your details based on your entry designs. And generally observe databases performance as you grow.
In short, the proper database is determined by your app’s composition, velocity desires, And just how you be expecting it to improve. Acquire time to choose correctly—it’ll preserve a great deal of problems later.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, each little delay adds up. Improperly published code or unoptimized queries can decelerate efficiency and overload your program. That’s why it’s crucial that you Create effective logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away everything needless. Don’t choose the most elaborate solution if a straightforward a single functions. Keep the features short, centered, and easy to check. Use profiling equipment to find bottlenecks—destinations where by your code normally takes as well extensive to run or uses an excessive amount memory.
Up coming, look at your databases queries. These frequently sluggish issues down much more than the code itself. Ensure that Each and every question only asks for the information you truly want. Avoid Decide on *, which fetches every thing, and in its place pick unique fields. Use indexes to speed up lookups. And keep away from undertaking too many joins, Specially throughout big tables.
When you discover precisely the same data getting asked for again and again, use caching. Retailer the final results briefly applying resources like Redis or Memcached and that means you don’t have to repeat pricey functions.
Also, batch your databases functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app extra effective.
Remember to examination with substantial datasets. Code and queries that work good with 100 information may possibly crash if they have to take care of one million.
In short, scalable apps are rapidly applications. Keep the code limited, your queries lean, and use caching when desired. These ways help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more end users plus much more website traffic. If all the things goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these instruments support maintain your application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of one server accomplishing the many get the job done, the load balancer routes end users to diverse servers depending on availability. This means no one server will get overloaded. If just one server goes down, the load balancer can ship traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing knowledge temporarily so it might be reused speedily. When consumers request the exact same information and facts once again—like a product site or possibly a profile—you don’t have to fetch it within the database every time. You may serve it within the cache.
There are 2 common forms of caching:
1. Server-aspect caching (like Redis or Memcached) outlets information in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces databases load, increases pace, and will make your app additional successful.
Use caching for things which don’t modify normally. And often be certain your cache is up to date when details does modify.
Briefly, load balancing and caching are easy but strong tools. Collectively, they assist your application deal with more end users, continue to be quick, and Recuperate from complications. If you plan to expand, you require each.
Use Cloud and Container Equipment
To make scalable applications, you will need instruments that permit your application develop very easily. That’s where by cloud platforms and containers are available in. They provide you overall flexibility, cut down set up time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you'll need them. You don’t must get components or guess long run capability. When targeted traffic boosts, you may insert extra means with just some clicks or automatically using auto-scaling. When traffic drops, you are able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and safety equipment. It is possible to center on building your application instead of running infrastructure.
Containers are A further vital Resource. A container deals your app and everything it really should operate—code, libraries, options—into 1 device. This can make it effortless to move your application involving environments, from a notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes enable you to control them. Kubernetes handles deployment, scaling, and Restoration. If just one element of your application crashes, it restarts it instantly.
Containers also make it very easy to individual elements of your application into companies. You are able to update or scale sections independently, which can be perfect for functionality and reliability.
Briefly, utilizing cloud and container applications implies you could scale rapidly, deploy easily, and Get well quickly when troubles happen. If you need your app to improve with out boundaries, begin employing these tools early. They preserve time, cut down danger, and allow you to stay focused on constructing, not correcting.
Keep track of Almost everything
If you don’t check your software, you received’t know when things go Improper. Checking assists you see how your application is accomplishing, spot concerns early, and make superior conclusions as your application grows. It’s a important Section of making scalable systems.
Begin by tracking standard metrics like CPU utilization, memory, disk House, and reaction time. These show you how your servers and services are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you collect and visualize this info.
Don’t just keep an eye on your servers—keep an eye on your application far too. Regulate how much time it's going to take for buyers to load internet pages, how frequently faults happen, and where they happen. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s happening inside your code.
Set up alerts for important problems. For example, if your reaction time goes over a limit or a service goes down, you'll want to get notified straight away. This allows you deal with difficulties rapidly, usually just before customers even notice.
Checking is likewise valuable once you make modifications. If you deploy a completely new attribute and see a spike in errors or slowdowns, you could roll it back again prior to it triggers real destruction.
As your app grows, visitors and details enhance. With out checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, monitoring will help you keep your application dependable and scalable. It’s not almost spotting failures—it’s about knowledge your program and ensuring that it really works nicely, even stressed.
Final Feelings
Scalability isn’t just for massive companies. Even modest applications want a solid foundation. By coming up with cautiously, optimizing correctly, and utilizing the proper applications, you are able to Make apps that expand effortlessly with out breaking Gustavo Woltmann news stressed. Get started little, Assume big, and Create good.